html表格表单使用小结
1.表格基础知识
- 1.1数据标签:
序号 | 标签 | 描述 |
---|---|---|
1 | <table> | 定义表格, 必选 |
2 | <tr> | 定义表格中的行, 必选 |
3 | <th> | 定义表格头部中的单元格, 必选 |
4 | <td> | 定义表格主体中的单元格, 必选 |
- 1.2结构标签:
序号 | 标签 | 描述 |
---|---|---|
1 | <option> | 定义表格标题, 可选 |
2 | <thead> | 定义表格头格, 只需定义一次, 可选 |
3 | <tbody> | 定义表格主体, 允许定义多次, 可选 |
4 | <tfooter> | 定义表格底, 只需定义一次, 可选 |
- 1.3常用属性:
序号 | 属性 | 所属标签 | 描述 |
---|---|---|---|
1 | border | <table> | 添加表格框 |
2 | cellpadding | <table> | 设置单元格内边距 |
2 | cellspacing | <table> | 设置单元格边框间隙 |
2 | align | 不限 | 设置单元格内容水平居中 |
2 | bgcolor | 不限 | 设置背景色 |
2.表格制作购物车实战页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>表格实战:购物车</title>
<style>
/* 设置表格字体大小 */
table {
font-size: 14px;
}
/* 将单元格之间间隙去掉 */
table {
border-collapse: collapse;
width: 70%;
margin: auto;
color: #888;
font-size: larger;
text-align: center;
}
td,
th {
border-bottom: 1px solid #ccc;
padding: 10px;
}
/* 标题样式 */
table caption {
font-size: 1.5rem;
color: yellowgreen;
margin-bottom: 20px;
}
table th {
font-weight: bolder;
color: white;
}
table thead tr:first-of-type {
background-color: teal;
}
/* 隔行变色 */
table tbody tr:nth-of-type(even) {
background-color: yellowgreen;
color: white;
}
/* 鼠标悬停效果 */
table tbody tr:hover {
background-color: violet;
color: black;
}
/* 表格底部样式 */
table tfoot td {
border-bottom: none;
color: red;
font-size: 1.2rem;
}
/* 结算按钮 */
body div:last-of-type {
width: 70%;
margin: 10px auto;
}
body div:first-of-type button {
/* 右浮动,靠右 */
float: right;
width: 120px;
height: 32px;
background-color: seagreen;
color: white;
border: none;
/* 设置鼠标样式 */
cursor: pointer;
}
body div:first-of-type button:hover {
background-color: sandybrown;
font-size: 1.1rem;
}
</style>
</head>
<body>
<!-- 表格 -->
<table>
<!-- 标题 -->
<caption>
购物车
</caption>
<!-- 表格头部 -->
<thead>
<tr>
<th>ID</th>
<th>品名</th>
<th>单价/元</th>
<th>单位</th>
<th>数量</th>
<th>金额/元</th>
</tr>
</thead>
<!-- 表格主体 -->
<tbody>
<tr>
<td>SN-1010</td>
<td>MacBook Pro电脑</td>
<td>18999</td>
<td>台</td>
<td>1</td>
<td>18999</td>
</tr>
<tr>
<td>SN-1020</td>
<td>iPhone手机</td>
<td>4999</td>
<td>部</td>
<td>2</td>
<td>9998</td>
</tr>
<tr>
<td>SN-1030</td>
<td>智能AI音箱</td>
<td>399</td>
<td>只</td>
<td>5</td>
<td>1995</td>
</tr>
<tr>
<td>SN-1040</td>
<td>SSD移动硬盘</td>
<td>888</td>
<td>个</td>
<td>2</td>
<td>1776</td>
</tr>
<tr>
<td>SN-1050</td>
<td>黄山毛峰</td>
<td>999</td>
<td>斤</td>
<td>3</td>
<td>2997</td>
</tr>
</tbody>
<!-- 表格尾部 -->
<tfoot>
<td colspan="4">总计:</td>
<td>13</td>
<td>35765</td>
</tfoot>
</table>
<!-- 结算按钮 -->
<div>
<button>结算</button>
</div>
</body>
</html>
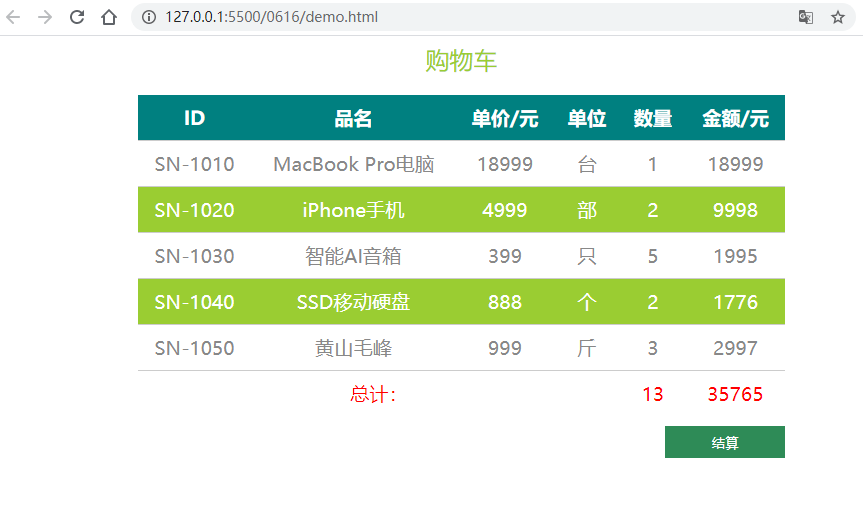
运行结果截图
- 换个姿势玩表格(div 模拟表格)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>换个姿势玩表格</title>
<style>
/* 表格 */
.table {
display: table;
border: 1px solid #000;
width: 300px;
text-align: center;
margin: auto;
}
/* 标题 */
.table-caption {
display: table-caption;
}
/* 表头 */
.table-thead {
display: table-header-group;
background-color: #ccc;
}
/* 行 */
.table-row {
display: table-row;
}
/* 列 */
.table-cell {
display: table-cell;
border: 1px solid #000;
padding: 5px;
}
/* 主体 */
.table-tbody {
display: table-row-group;
}
/* 底部样式 */
.table-tfoot {
display: table-footer-group;
}
/* 列分组样式 */
.table-colgroup {
display: table-column-group;
}
.table-colgroup .table-col:first-of-type {
display: table-column;
background-color: sandybrown;
}
.table-colgroup .table-col {
display: table-column;
background-color: seagreen;
}
</style>
</head>
<body>
<!-- 表格table -->
<div class="table">
<!-- 标题<caption> -->
<div class="table-caption">
员工信息表
</div>
<!-- 列分组<colgroup> -->
<div class="table-colgroup">
<div class="table-col"></div>
<div class="table-col"></div>
<div class="table-col"></div>
</div>
<!-- 表头<thead> -->
<div class="table-thead">
<!-- 行 -->
<div class="table-row">
<div class="table-cell">ID</div>
<div class="table-cell">姓名</div>
<div class="table-cell">职务</div>
</div>
</div>
<!-- 表格主体 -->
<div class="table-tbody">
<div class="table-row">
<div class="table-cell">1</div>
<div class="table-cell">小王</div>
<div class="table-cell">程序员</div>
</div>
<div class="table-row">
<div class="table-cell">2</div>
<div class="table-cell">小张</div>
<div class="table-cell">组长</div>
</div>
<div class="table-row">
<div class="table-cell">2</div>
<div class="table-cell">小李</div>
<div class="table-cell">程序员</div>
</div>
<div class="table-row">
<div class="table-cell">3</div>
<div class="table-cell">小朱</div>
<div class="table-cell">组长</div>
</div>
</div>
<!-- 表格尾部<tfoot> -->
<div class="table-tfoot">
<div class="table-row">
<div class="table-cell">x</div>
<div class="table-cell">y</div>
<div class="table-cell">z</div>
</div>
</div>
</div>
</body>
</html>
运行结果截图(div 布局表格)
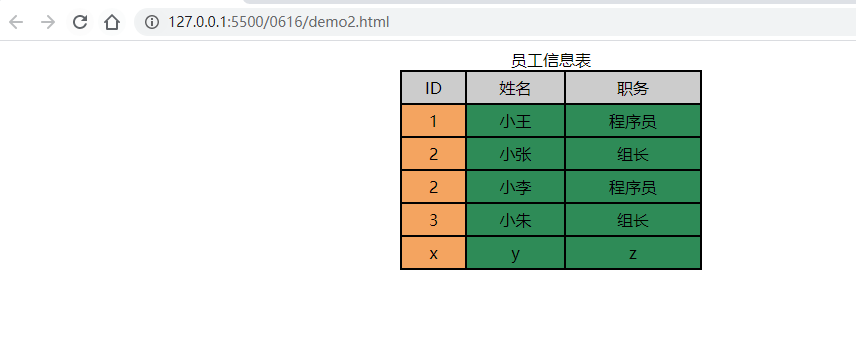
3.表单
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>基本表单元素</title>
<style>
body {
color: #555;
}
h3 {
text-align: center;
}
form {
width: 450px;
margin: 30px auto;
border-top: 1px solid #aaa;
}
form fieldset {
border: 1px solid seagreen;
background-color: lightcyan;
box-shadow: 2px 2px 4px #bbb;
border-radius: 10px;
margin: 20px;
}
form fieldset legend {
background-color: rgb(178, 231, 201);
border-radius: 10px;
color: seagreen;
padding: 5px 15px;
}
form div {
margin: 5px;
}
form p {
text-align: center;
}
form .btn {
width: 80px;
height: 30px;
border: none;
background-color: seagreen;
color: #ddd;
}
form .btn:hover {
background-color: coral;
color: white;
cursor: pointer;
}
input:focus {
background-color: rgb(226, 226, 175);
}
</style>
</head>
<body>
<h3>用户注册</h3>
<!-- form+input -->
<form action="" method="POST">
<fieldset>
<legend>基本信息(必填)</legend>
<div>
<label for="username">账号:</label>
<input
type="text"
id="my-username"
name="username"
placeholder="6-15位字符"
/>
</div>
<div>
<label for="email-id">邮箱:</label>
<input
type="email"
name="email"
id="email-id"
placeholder="demo@qq.com"
required
/>
</div>
<!-- 密码 框-->
<div>
<label for="pwd-1">密码:</label>
<input
type="password"
name="password1"
id="pwd-2"
placeholder="不少于6位且字母+数字"
/>
<!-- 点击显示密码 -->
<!-- <button onclick="ShowPsw()" id="btn" type="button">显示密码</button> -->
</div>
<div>
<label for="pwd-2">确认:</label>
<input type="password" name="password2" id="pwd-1" />
</div>
</fieldset>
<fieldset>
<legend>扩展信息(选填)</legend>
<div>
<label
>生日:
<input type="date" name="birthday" />
</label>
</div>
<!-- 单选按钮 -->
<div>
<label for="secret">性别:</label>
<!-- 单选按钮中在name属性名必须相同 -->
<input type="radio" value="male" name="gender" id="male" /><label
for=""
>男</label
>
<input type="radio" value="female" name="gender" id="female" /><label
for=""
>女</label
>
<input
type="radio"
value="secret"
name="gender"
id="secret"
checked
/><label for="secret">保密</label>
</div>
<!-- 复选框 -->
<div>
<label for="programme">爱好</label>
<!-- 因为复选框返回是一个或多个值,最方便后端用数组来处理, 所以将name名称设置为数组形式便于后端脚本处理 -->
<input type="checkbox" name="hobby[]" id="game" value="game" />
<label for="game">打游戏</label>
<input type="checkbox" name="hobby[]" id="shoot" value="shoot" />
<label for="game">编程</label>
<input
type="checkbox"
name="hobby[]"
id="programme"
value="programme"
checked
/>
<label for="game">编程</label>
</div>
<!-- 选项列表 -->
<div>
<label for="brand">手机:</label>
<input type="search" list="phone" id="brand" name="brand" />
<datalist id="phone">
<option value="apple"> </option>
<option value="huawei">华为</option>
<option value="mi" label="小米"> </option>
</datalist>
</div>
</fieldset>
<fieldset>
<legend>其它信息(选填)</legend>
<!--文件上传-->
<div>
<label for="uploads">上传头像:</label>
<input
type="file"
name="user_pic"
id="uploads"
accept="image/png, image/jpeg, image/gif"
/>
</div>
<!--文本域-->
<div>
<label for="resume">简历:</label>
<!--注意文本域没有value属性-->
<textarea
name="resume"
id="resume"
cols="30"
rows="5"
placeholder="不超过100字"
></textarea>
</div>
</fieldset>
<!-- 隐藏域, 例如用户的Id, 注册,登录的时间,无需用户填写 -->
<input type="hidden" name="user_id" value="123" />
<p>
<input type="submit" value="提交" class="btn" />
<button class="btn">提交</button>
</p>
</form>
<script>
//输入密码,点击名文显示
// function ShowPsw(ele) {
// const pwd = document.querySelector("#pwd-2");
// pwd.type = "text";
// 代码写成一行
// document.querySelector("#pwd-2").type = "text";
// }
</script>
</body>
</html>
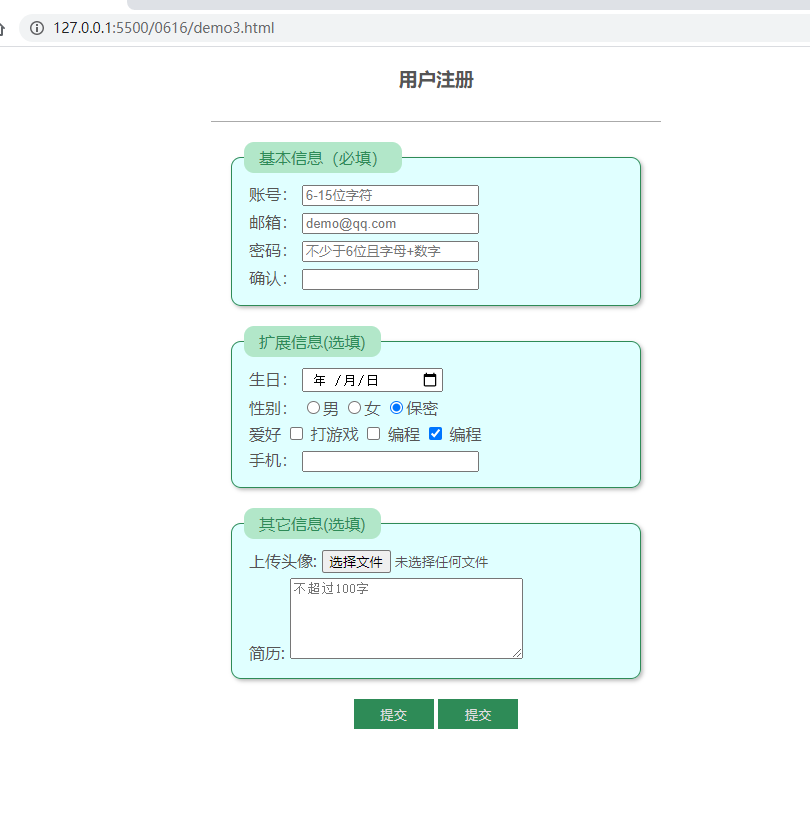
4.总结
- 表格是数据格式化的工具
- 完整表格 9 个标签:( table + caption + colgroup + thead + tbody + tfoot + tr + th + td)
- 表格常用 7 个标签:table+capiton+thead+tbody+tr+th+tr+td
- 最简单表格要用的 6 个标签:table+caption+tbody+tr+th/td
- 表单是网站页面与用户之间实现互动一种工具,通常用来实现用户的注册登陆,信息收集
- 表单元素添加
disabled
禁用属性的字段数据不会被提交,但是readonly
只读属性的字段允许提交 - 表单元素隐藏域的内容不会在 HTML 页面中显示,但是
value
属性的数据会被提交 - 4.1 表格
<table></table>:表格
<caption></caption>:表格标题
<thead></thead>:表格头部
<tr></tr>:表示行
<th></th>:表格头部单元格
<tbody></tbody>:表格主体内容
<td></td>:单元格
<tfoot></tfoot>:表格尾部内容
- 4.2 表格另一种表格形式
- 用 css 样式与 div 结合来表示
.table:就表示<table>标签,并设置display:table
.table-caption:就表示<caption>标签,并设置display:table-caption
.table-thead:就表示<thead>标签,并设置display:table-header-group
.table-row:就表示<tr>标签,并设置display:table-row
.table-cell:就表示单元格,并设置display:table-cell
.table-tbody:就表示<tbody>标签,并设置display:table-row-group
.table-tfoot:就表示<tfoot>标签,并设置display:table-footer-group
.table-colgroup:表示列分组样式,设置display: table-column-group;
- 4.3 表单
<form action="跳转地址" method="get/post">表单内容</form>
<fieldset><legend>信息</legend></fieldset>: 控件组
<label for="#id">名称:</label>:绑定到input标签
<input type="text" id="" name="变量名称" placeholder="占位符" autofocuse required/> 文本框
autofocuse输入框默认显示光标,required提交时对输入框内容进行判断是否符合要求
<input type="password" id="" name="变量名称" placeholder="占位符"> 密码框
<input type="email" id="" name="" /> 邮箱
<input type="radio" id="" name="name" value="" /> 单选框
<input type="radio" id="" name="name" value="" /> 单选框
radio属性name必须是相同的
<input type="checkbox" id="" name="name[]" value="" /> 多选框
因为复选框返回的是一个值或多个值,最方便用后端数组来处理,所有将name名称设置为数组形式便于后端脚本处理
<input type="file" id="" name="" accept="image/png,image/jpeg" />上传文件
<input type="search" list="" name="name" />选项列表
选项列表与datalist绑定<datalist id="name"><option value=""></option></datalist>
<textarea name="" id="" rows="" cols=""></textarea>多行文本框
<input type="hidden" name="" value="" />隐藏域,用于提交用户信息,登录时间
<input type="submit" value="提交" />提交按钮,用于向服务端提交数据